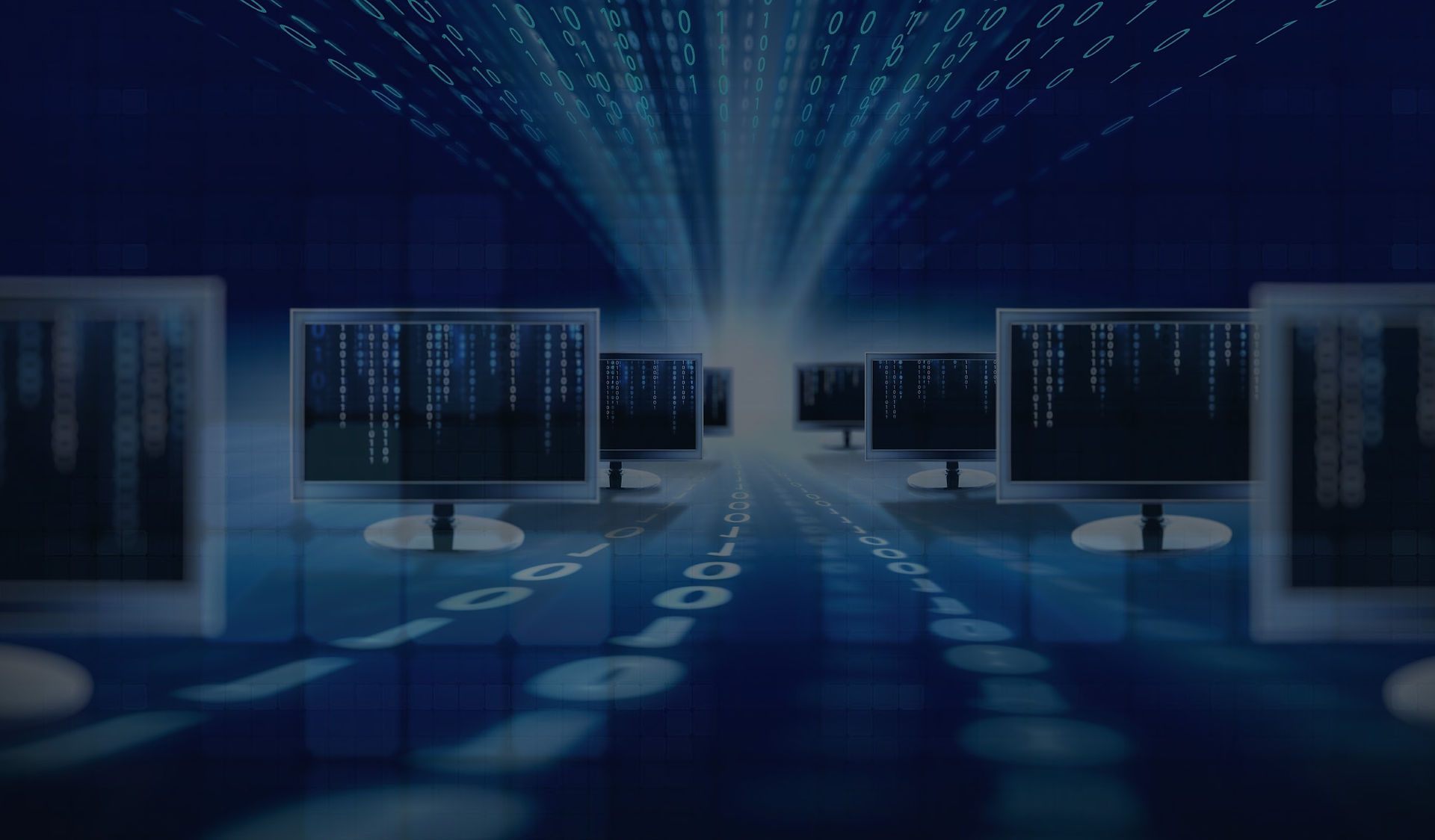
SOFTWARE

ENGINEERING
Activity based learning ...
TESTING (JUnit)
Objectives
-
To do unit testing of the project code
-
To perform exhaustive white box testing
-
To understand test coverage criterion
Description
In software engineering, a walkthrough is a form of software peer review in which a designer or programmer leads members of the development team and other interested parties through a software product, and the participants ask questions and make comments about possible errors, violation of development standards, and other problems.
Software testing is the execution of the software with actual test data. Exhaustive testing is the execution of every possible test case. A test coverage criterion is a rule about how to select tests and when to stop testing. The standard approach is to use the subsumes relationship. Once a programmer has written the code for a module, it has to be verified before it is used by others. Testing remains the most common method of this verification. At the programmer level the testing done for checking the code the programmer has developed (as compared to checking the entire software system) is called unit testing.
Tools
-
JUnit: JUnit is a simple framework to write repeatable tests. It is an instance of the xUnit architecture for unit testing frameworks.
-
NUnit: NUnit is a unit-testing framework for all .Net languages. Initially ported from JUnit, the current production release, version 2.6, is the seventh major release of this xUnit based unit testing tool for Microsoft .NET. It is written entirely in C# and has been completely redesigned to take advantage of many .NET language features, for example custom attributes and other reflection related capabilities. NUnit brings xUnit to all .NET languages.
-
CUnit: CUnit is a lightweight system for writing, administering, and running unit tests in C. It provides C programmers a basic testing functionality with a flexible variety of user interfaces.
Procedure
-
Unit testing: It is like regular testing where programs are executed with some test cases except that the focus is on testing smaller programs or modules which are typically assigned to one programmer (or a pair) for coding. In the programming processes we discussed earlier, the testing was essentially unit testing. A unit may be a function or a small collection of functions for procedural languages, or a class or a small collection of classes for object-oriented languages.
-
Functional testing: In functional testing, the specification of the software is used to identify subdomains that should be tested. One of the first steps is to generate a test case for every distinct type of output of the program.
-
Test matrices: A way to formalize this identification of subdomains is to build a matrix using the conditions that we can identify from the specification and then to systematically identify all combinations of these conditions as being true or false.
-
Structural testing: Structural testing is based on the structure of the source code. The simplest structural testing criterion is every statement coverage, often called C0 coverage.
-
C0 coverage: This criterion is that every statement of the source code should be executed by some test case. The normal approach to achieving C0 coverage is to select test cases until a coverage tool indicates that all statements in the code have been executed.
-
C1-Every-branch testing: A more thorough test criterion is every-branch testing, which is often called C1 test coverage. In this criterion, the goal is to go both ways out of every decision.
-
Every-path testing: Even more thorough is the every-pathtesting criterion. A path is a unique sequence of program nodes that are executed by a test case.
-
Multiple-condition coverage: A multiple-condition testing criterion requires that each primitive relation condition is evaluated both true and false.
-
Subdomain testing: Subdomain testing is the idea of partitioning the input domain into mutually exclusive subdomains and requiring an equal number of test cases from each subdomain.
-
-
Data flow testing: Data flow testing is testing based on the flow of data through a program.
-
Random testing: Random testing is accomplished by randomly selecting the test cases. This approach has the advantage of being fast and it also eliminates biases of the testers.
-
Boundary testing: Boundary testing is aimed at ensuring that the actual boundary between two subdomains is as close as possible to the specified boundary.
Problem
Write a working code for any one of the modules in a language of your choice. Adhere to coding standards (Annexure III)
Test the classes by creating objects of those classes. Take the object to a particular state, invoke a method on it, and then check whether the state of the object is as expected. This sequence has to be executed many times for a method, and has to be performed for all the methods. Use any of the tools mentioned in the list.
For the software that you have developed, identify the subdomains that should be tested. Build a matrix using the conditions that you can identify from the specification and then to systematically identify all combinations of these conditions as being true or false. (Annexure IV). Show the following:
-
C0 coverage: Every statement coverage
-
C1 coverage: Every branch testing & every path testing
-
Multiple-condtion coverage
-
Subdomain testing
Draw a control flow graph showing the data flow of your code. Also perform random testing and boundary testing.
Deliverables
Error-free code
References
-
David Gustafson: Software Engineering, Schaum's Outline Series, McGraw Hill, 2002, pg. 136-152
-
Roger S. Pressman: Software Engineering A Practitioner's Approach, 7th Edition, McGraw Hill, 2010, pg. 180-181